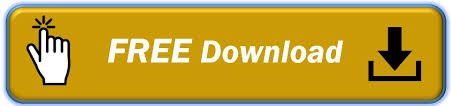
LinkedList doesn't have an array but a double-ended queue of mutually-connected elements instead. If an element is removed, the size is decreased. If an element is added, the size is increased. This means that ArrayList internally contains an array of values and a counter variable to know the current size at any point.
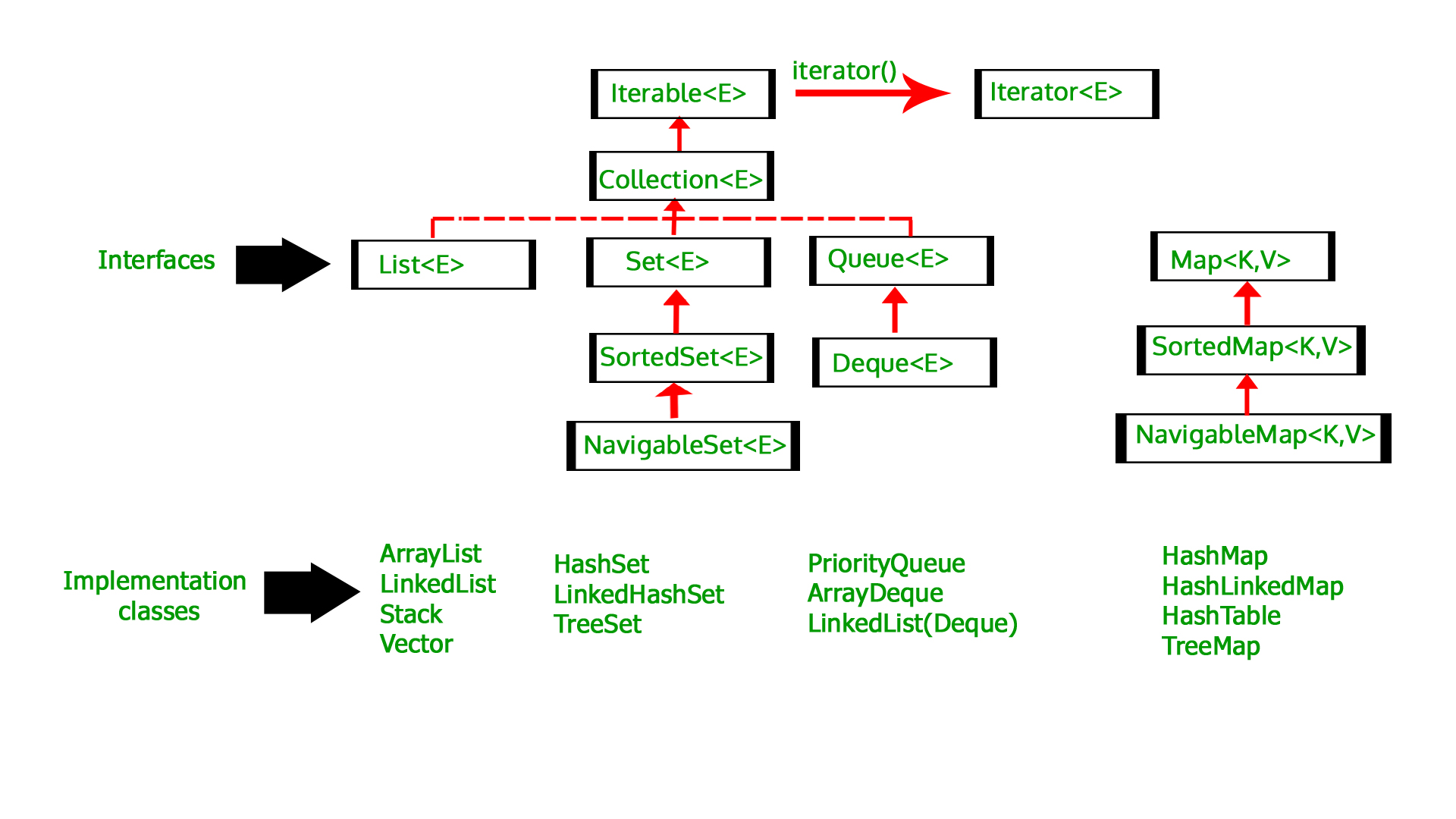
A LinkedList is a doubly-linked list/queue implementation. Inner Workings of ArrayList and LinkedListĪn ArrayList is a resizable array that grows as additional elements are added. However, the LinkedList also implements the Queue interface. Since it's an interface, it simply provides a list of methods that need to be overridden in the actual implementation class.ĪrrayList and LinkedList are two different implementations of these methods. In Java, List is an interface under the java.util package. Lists oftentimes go hand in hand with other mechanisms such as Java Streams which offer simple yet effective ways for iteration, filtering, mapping, and other useful operations. They are convenient because they enable easy manipulation of elements (such as insertion or fetching) and simple iteration of the entire collection. Lists are therefore ordered collections (unlike sets) which also allow duplicates. This means that each element of the list has both a predecessor and a successor (except the first and the last, of course - they only have one of each). Lists are data structures used for sequential element storage. Knowing which implementation of a List to use in which situation is an essential skill. In this article, we'll go through both of these implementations, observe their inner workings and discuss their performance. Should you choose an ArrayList or a LinkedList? What's the difference between these two? In Java, a common question when using a List implementation is: " & Format(finish.Millisecond, " 000"))Ĭonsole.Lists are some of the most commonly used data structures. " & ltimer.Millisecond)Ĭonsole.WriteLine( " Finish Time = " & finish.Second & ". Ltime = (finish.Second - ltimer.Second) * 1000 + finish.Millisecond - ltimer.MillisecondĬonsole.WriteLine( " Start Time = " & ltimer.Second & ". " & atimer.Millisecond)Ĭonsole.WriteLine( " Array Time = " & atime.ToString)

" & finish.Millisecond)Ĭonsole.WriteLine( " Array List Time = " & altime)Ītime = (finish.Second - atimer.Second) * 1000 + finish.Millisecond - atimer.MillisecondĬonsole.WriteLine( " Start Time = " & atimer.Second & ". " & altimer.Millisecond)Ĭonsole.WriteLine( " Finish Time = " & finish.Second & ". From the Collection of Dictionaries down to this does a LOT.Īltime = (finish.Second - altimer.Second) * 1000 + finish.Millisecond - altimer.MillisecondĬonsole.WriteLine( " Start Time = " & altimer.Second & ".
#Array vs arraylist picture code#
Think for a minute what that is doing, it's iterating through a Collection of Dictionary Collections and getting the appropriate string for each and very very rapidly and efficiently! // Follow the code path through that down to this other one above and you will see what I mean. Take the first one: // var x = listOfLists.Aggregate blah blah. Aggregate here are good examples and it can be many Types. " \n\nWhich is set to the Data file: \n" + " The following currently use this Reports Folder:\n\n" ĭic.Aggregate(text, (current, pair) => current + var i = 0 // var text = "" // foreach (var dic in listOfLists) // \n\n", dir), / /// private static void DisplayResults / & faster because instead of a loop it's a LINQ query! / It directly calls the Mother Method of them all below this one.

/ ViewAllAssociations() Has it's own special Size & Location Settings. / This one accepts the Collection of Dictionaries for Here are a couple of prime examples in optimized code form. If you do not exceed the capacity it is going to be as fast as an array.īetter handling and much easier way of doing things.Ĭopy Code // The main thing I can add to this is that a LINQ query is almost always faster than a loop such as a foreach, etc. However because ArrayList uses an Array is faster to search O(1) in it than normal lists O(n). Since the add from ArrayList is O(n) and the add to the Array is O(1).
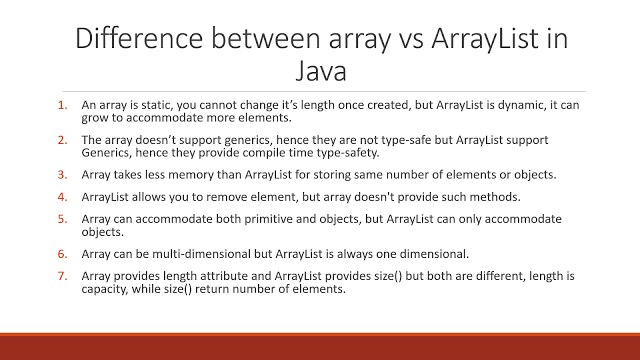
You will only feel this if you add to often. It creates a new Array and copies every element from the old one to the new one. However when you add an element to the ArrayList and it overflows. Where as, the lower bound of an ArrayList is always zero.Īrray is faster and that is because ArrayList uses a fixed amount of array. Where as, ArrayList always has exactly one dimension. Where as, ArrayList is in the System.Collections namespace. Where as, ArrayList can hold item of different types. Where as, ArrayList can increase and decrease size dynamically.Īn Array is a collection of similar items.
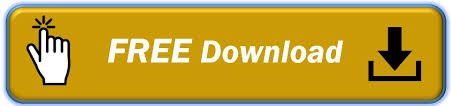